Difference Between Primitives And Wrappers
1. Short answer
The key differences between primitives and wrappers in Java are:
- Type: Primitives are basic data types (e.g.,
int
,char
), while wrappers are objects (e.g.,Integer
,Character
) that represent primitive types. - Memory: Primitives are stored directly in memory (on the stack), whereas wrappers are objects stored in heap memory, consuming more space.
- Nullability: Primitives cannot be
null
, but wrapper objects can be, making them helpful in representing absent values. - Usage in Collections: Primitives cannot be used in collections (like
ArrayList
), but wrappers can. - Performance: Primitives are faster and more memory-efficient, while wrappers offer additional functionality (e.g., utility methods) at the cost of performance.
In short, primitives are more efficient for performance-critical operations, while wrappers are needed for working with objects and collections.
2. Long answer
In Java, data is typically represented using two main types: primitive types and wrapper classes. These two categories serve similar purposes in that they store values, but they have key differences that can affect performance, functionality, and usage in various contexts. Understanding the distinction between these types is crucial for optimizing code and ensuring proper usage in different scenarios.
Primitive Types
Primitive types are the most basic data types in Java. They are not objects, and they represent raw values. Java provides 8 primitive types:
Hence, the 8 primitive data types in Java are categorized into four types.
- Integer types -
byte
,short
,int
, andlong
. - Floating point types -
float
anddouble
. - Character type -
char
. - Boolean type -
boolean
.
Primitive types are highly efficient because they represent raw values directly in memory. They are stored in stack memory, which makes them faster and less resource-intensive compared to objects. However, they come with certain limitations, such as the inability to be used in generic types and collections like ArrayList
, which require objects.
Wrapper Classes
Wrapper classes in Java are part of the java.lang
package and are used to convert primitive data types into objects. Each primitive type has a corresponding wrapper class:
- Byte for byte
- Short for short
- Integer for int
- Long for long
- Float for float
- Double for double
- Character for char
- Boolean for boolean
Wrapper classes enable primitives to be treated as objects. This allows primitives to be used in situations that require objects, such as in collections (e.g., ArrayList
, HashMap
), or when dealing with APIs that require objects rather than primitive types. The wrapper classes also provide utility methods like parseInt()
or valueOf()
for converting strings to primitives, and methods like toString()
to convert primitives to string representations.
Key Differences Between Primitives and Wrappers
- Memory -> Primitives are stored directly in memory (typically stack memory), while wrapper classes are stored as objects in heap memory. As a result, wrappers consume more memory due to object overhead.
- Nullability -> Primitive types cannot be assigned
null
because they always have a default value (e.g.,0
forint
,false
forboolean
). Wrapper classes, however, can be assignednull
, which can be useful in certain scenarios (e.g., when representing the absence of a value). - Performance -> Primitive types are faster and more efficient than wrapper classes because they don’t involve the overhead of object creation or garbage collection. However, wrappers offer more functionality at the cost of performance.
- Usage in Collections -> Since Java collections (like
ArrayList
,HashMap
, etc.) only accept objects, primitive types cannot be directly used in these collections. You must use wrapper classes to store primitive data in a collection. - Autoboxing and Unboxing -> are key features of Java that simplifies the use of primitive types and wrapper classes is autoboxing and unboxing. Autoboxing automatically converts a primitive type into its corresponding wrapper class, and unboxing converts a wrapper class back into the primitive type.
Autoboxing and unboxing example:
int num = 5;
Integer wrapperNum = num; // Autoboxing
int unboxedNum = wrapperNum; // Unboxing
This feature makes it easier to work with both primitives and wrappers without having to perform conversions manually.
Finally, the main difference between primitive types and wrapper classes lies in their nature and how they are used.
- Primitives are lightweight and efficient and are used in performance-critical applications. Wrapper classes, on the other hand, are helpful when objects are required, such as in collections, and they offer additional functionality at the cost of performance.
- Understanding when to use primitives versus wrappers is crucial for writing efficient and functional Java code, especially in contexts like interview preparation, where these concepts are often tested.
📎 Reference Materials
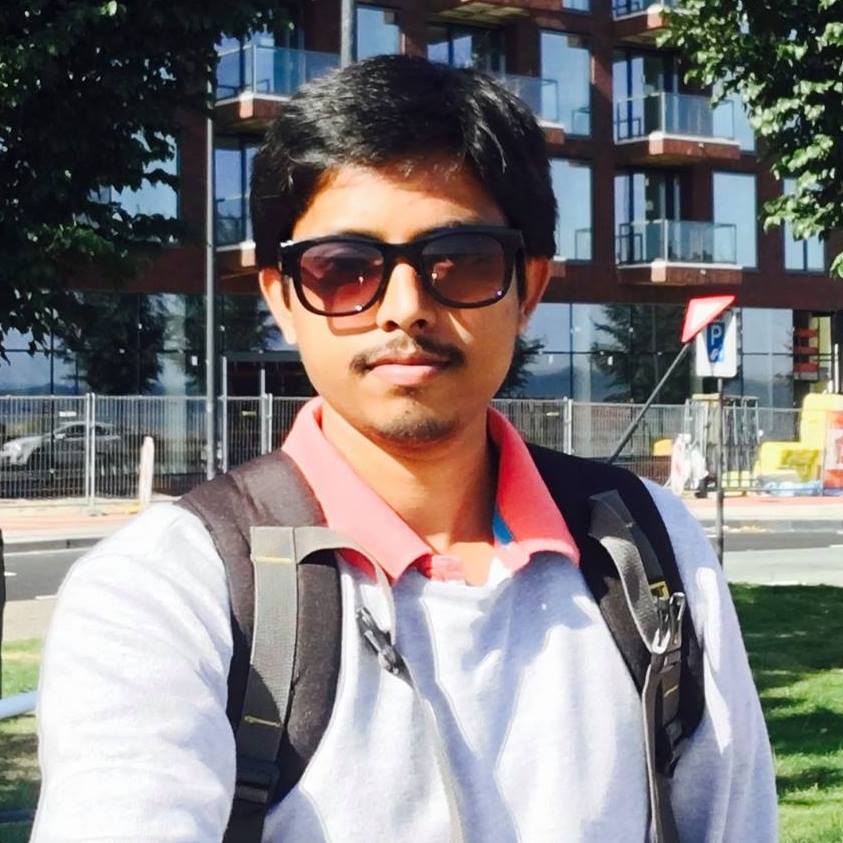
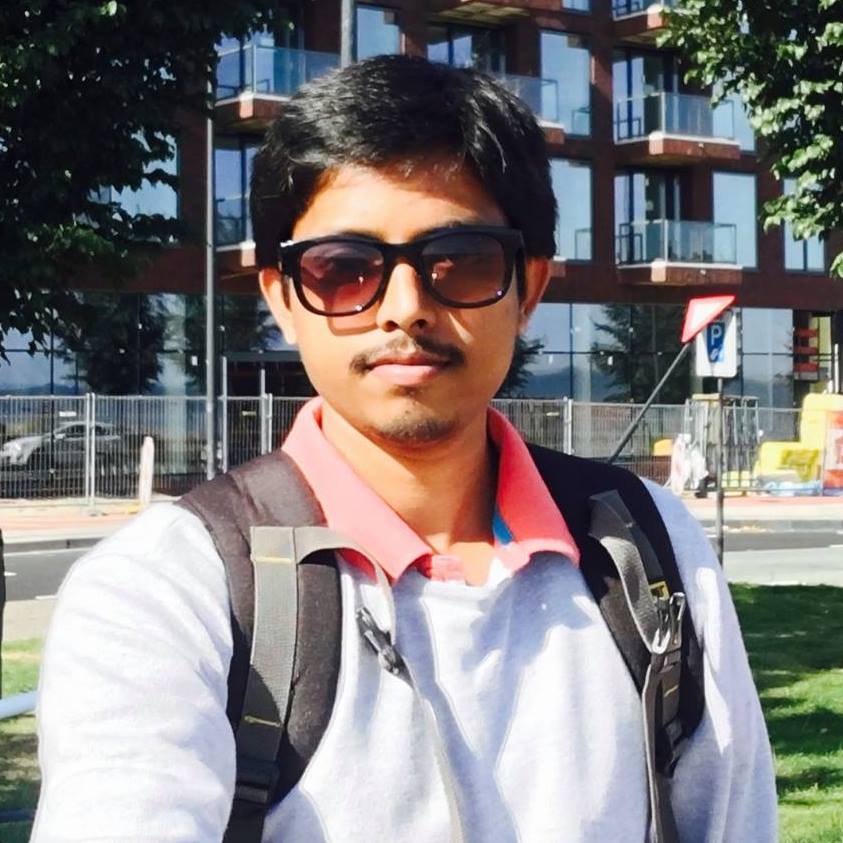