Access 2D Arrays
Problem Statement
You are given a m x n
integer matrix matrix
. Print all the elements in order.
Example#1:
Input: A = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
Output: 1, 2, 3, 4, 5, 6, 7, 8, 9
Example#2:
Input: A = {{10, 9, 4}, {34, 0, 1}, {9, 8, 9}};
Output: 10, 9, 4, 34, 0, 1, 9, 8, 9
Illustration
We must follow this traversal to print the elements in a specific order.
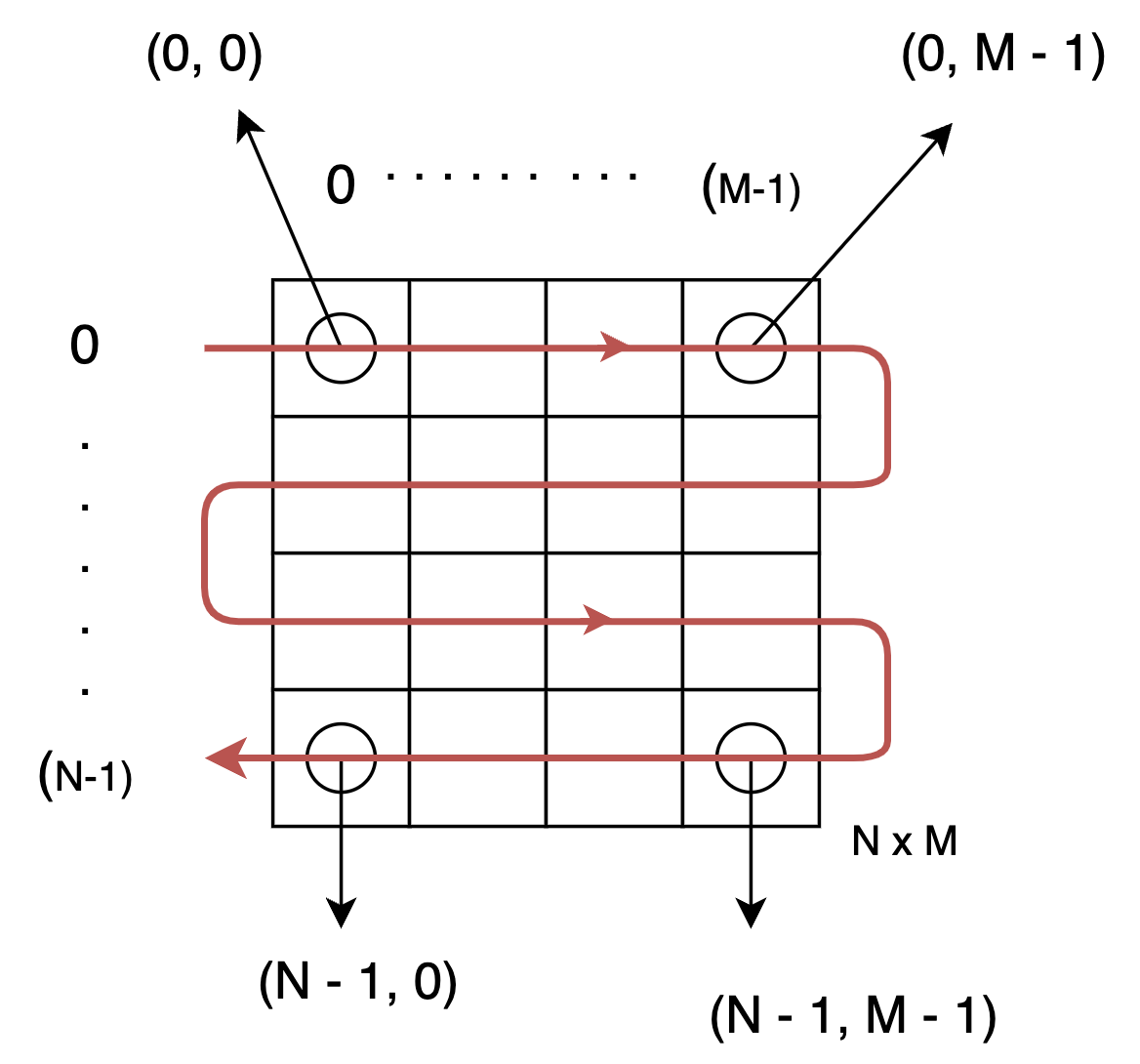
Intuition
This is an example problem that is too easy. There are different ways to solve this problem.
- Traditional for-loop.
- Using
deepToString
method. - Using 1D arrays
Traditional for-loop
We use two for-loops to loop through each row and column.
Code
public class PrintElements {
public static void main(String[] args) {
int[][] grid = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
usingForLoop(grid);
}
private static void usingForLoop(int[][] grid) {
int N = grid.length; // rows
int M = grid[0].length; // columns
for (int row = 0; row < N; row += 1) {
for (int col = 0; col < M; col += 1) {
System.out.println(grid[row][col]);
}
}
}
}
Another simple way to achieve this without N
and M
variables are:
for (int row=0; row < grid.length; row++)
{
for (int col=0; col < grid[row].length; col++)
{
int value = grid[row][col];
// Do stuff
}
}
Explanation
As you have seen in the illustration, there are N
rows and M
columns.
N
rows and M
columns can be found using the following:
- When
N == M
, we can sayN = M = grid.length
. - When
N != M
, which means the number of rows and columns is not equal. In this case, we findN
usinggrid.length
andM
columns usinggrid[0].length
.
So, for each row & column, we need to find the corresponding element using grid[row][col]
.
Complexity analysis
- Time complexity:
O(N x M)
: We must traverse through all the elements in each row and column. This is directly proportional toN
timesM
. IfN == M
, then we have aO(N^2)
complexity. - Space complexity -
O(1)
- No algorithmic memory is used here. We just used the inputA[][]
memory, hence Constant time.
Using deepToString method
This comes with Java API and is part of java.util
package.
import java.util.Arrays;
public class PrintElements {
public static void main(String[] args) {
int[][] grid = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
usingDeepToString(grid);
}
public static void usingDeepToString(int[][] grid) {
System.out.println(Arrays.deepToString(grid));
}
}
Above code time and space, complexity is the same; the deepToString
method from Java API does the same as the traditional loop.
Using 1D arrays
This is a rather straightforward approach using a for-each loop.
import java.util.Arrays;
public class PrintElements {
public static void main(String[] args) {
int[][] grid = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
forEachLoop(grid);
}
public static void forEachLoop(int[][] grid) {
// Note the different use of "row" as a variable name! This
// is the *whole* row, not the row *number*.
for (int[] row : grid) {
for (int value : row) {
System.out.println(value);
}
}
}
}
These above are merely examples of how to iterate a 2D array.