Execution/Test Class
Let us create an array with capacity
as 10
and insert 5
numbers in it, the size
of the array becomes 5
.
Illustration's
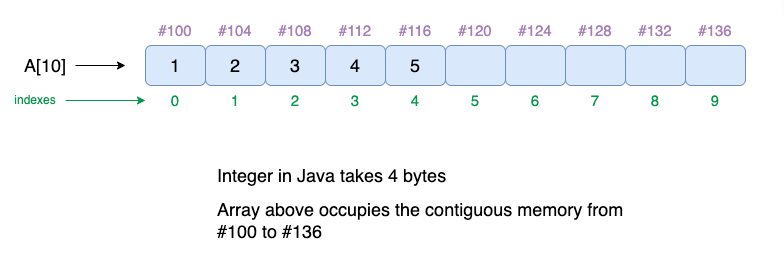
Another illustration that clarifies the difference between capacity
and size
of the array.
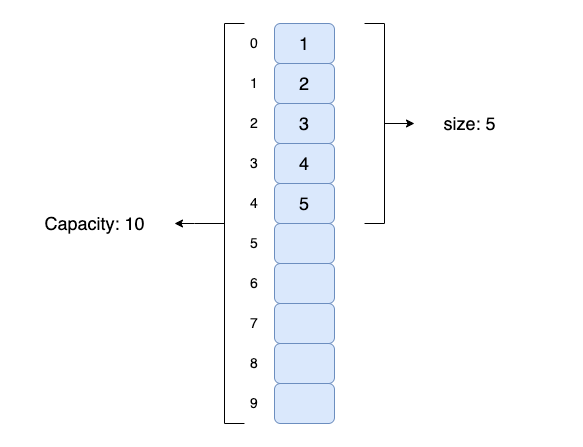
What do you think is stored in the index 5 to index 9? The answer is zeros.
So 0
's are stored from the index 5
to 9
. So when you access A[6]
you get 0
, for A[2]
you get 3
, and so on.
In the above, we have constructed an Array
class and basic operations insert
, remove
, get
, and size
etc. Let us test each of the methods to ensure nothing is breaking in our code.
Code
Following is the execution class
package dev.ggorantala.ds.arrays;
public class ArrayExecution {
private static final int[] INPUT = new int[] {1, 2, 3, 4, 5};
public static void main(String[] args) {
Array array = new Array(10);
for (int value : INPUT) {
array.insert(value);
}
array.remove(2); // removed element `3` from array
System.out.println(array.get(0)); // 1
System.out.println(array.size()); // 4
// The extra o's are because of the array capacity which is 10
array.print(); // 1, 2, 4, 5, 0, 0, 0, 0, 0, 0
}
}
Explanation
Array array = new Array(10);
creates an array with a capacity 10
.
The input array we declared holds 5
elements.
private static final int[] INPUT = new int[] {1, 2, 3, 4, 5};
Using a simple for-loop, we are storing the INPUT
data into our newly created array
instance variable.
for (int value : INPUT) {
array.insert(value);
}
We removed an element at the index 2
using array.remove(2);
Finally, we called get
, size
and print
methods to display data on the console.
Hurrah! You just implemented your first data structure in computer science and successfully tested it.