Overview Of Arrays
In this lesson, you will learn about arrays, their declaration and initialization, and how they are represented in RAM, with illustrations and code snippets.
Arrays are built in most programming languages. They are the most fundamental data structures in computer science and the building blocks for many other, more complex data structures.
In Java and many other languages, arrays are static(fixed size). Array organizes items sequentially, one after another, in memory.
The items could be Integer
, String
, Object
, – anything. The items are stored in contiguous (adjacent to each other) memory locations.
Each position in the array has an index, starting at the 0
th index. In Java, integers take 4
bytes, so the memory addresses of each adjacent element are added by 4
bytes.
Illustration
A simple sketch of this is as follows.
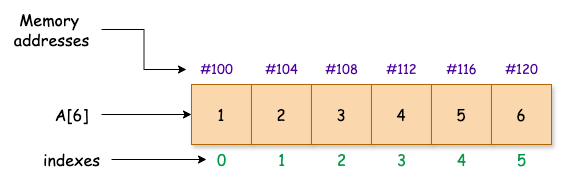
In above figure 1: Integer in Java takes4
bytes. Array occupies the contiguous memory from#100
to#120
If we say our array memory, location/address starts from 100
, then the following integer address will start from 104(100+4)
bytes, and so on.
Figure 1 contains an array with 6
elements in it. So, theoretically, anything that we store after this array takes the address from 124
.
Important Note: In Java, we have to specify the size of the array ahead of time before initializing the array.
We knew everything on the computer is stored in bits 0
or 1
.
Let's explore how "Figure 1" can be represented in memory and addressed using binary.
RAM is a storage device viewed as shelves containing memory locations following figure 2 is just a mere zoomed version of how data is stored inside RAM.
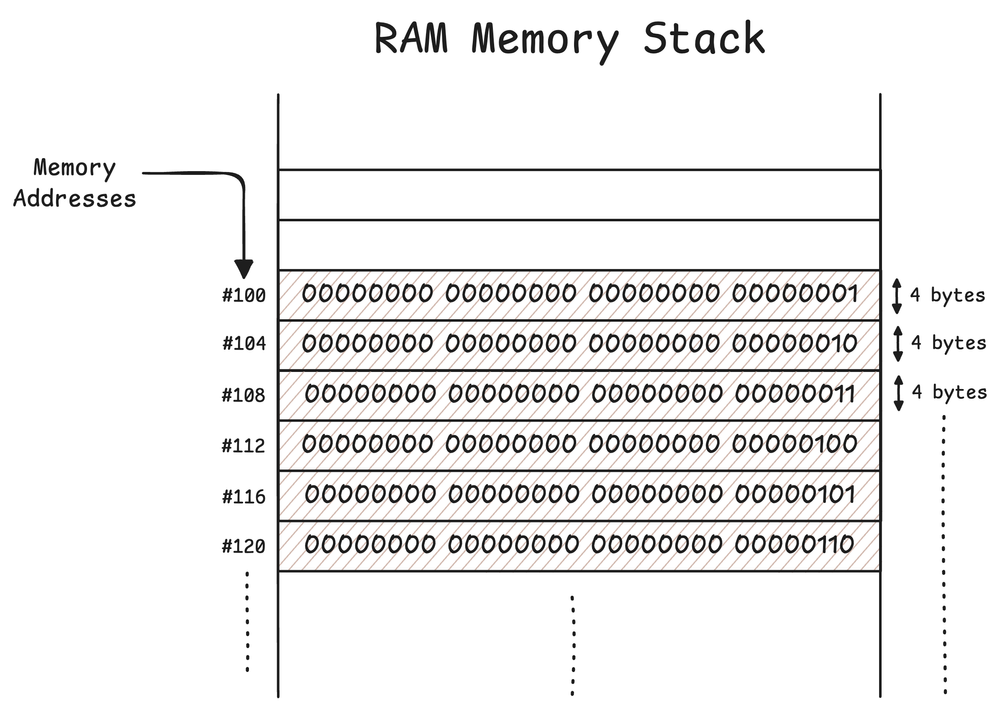
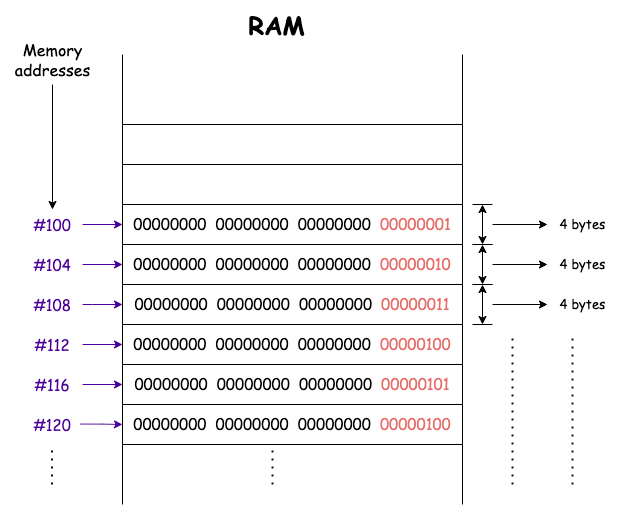
Figure 2 represents array values with memory locations in RAM shelves ranging from #100
to #120
. So what are all the 0
's and 1
's in the illustration? 🤔
They are 32-bit binary representations of decimal values. The following table represents binary values for each corresponding decimal value.
Decimal Number | 32-bit Binary Representation |
---|---|
1 | 00000000 00000000 00000000 00000001 |
2 | 00000000 00000000 00000000 00000010 |
3 | 00000000 00000000 00000000 00000011 |
4 | 00000000 00000000 00000000 00000100 |
5 | 00000000 00000000 00000000 00000101 |
6 | 00000000 00000000 00000000 00000110 |
Declaration and initialization
Consider an array A[]
that has 5
elements. To access the last element of the array, we use A[4]
.
With this knowledge, if N
is the array length, then (N-1)
is how we access the last element. There are two ways we can declare and initialize the array in Java.
What happens if we declare an array as follows?
int[] A = new int[3];
// stores 3 items, capacity = 3 and size is 0(no items added, so far)
System.out.println(Arrays.toString(A)); // [0, 0, 0]
Initially, we did not add any items to the array, so the array values are defaulted to 0
as seen above.
Let us see another way to declare and initialize the array.
// approach 1
int[] A = new int[5];
A[0] = 1;
A[1] = 2;
A[2] = 3;
A[3] = 4;
A[4] = 5;
// approach 2
int[] A = {1, 2, 3, 4, 5};
Arrays with char
datatype and String
class is as follows.
// String arrays
String[] fruits = new String[3]; // contains 3 strings
// char arrays
char[] chars = new char[256]; // contains 256 items
This small illustration helps you understand how we access array elements using their indexes.
How do you access elements?
Following is a simple sketch of an array A
with a capacity N
.
int[] A;
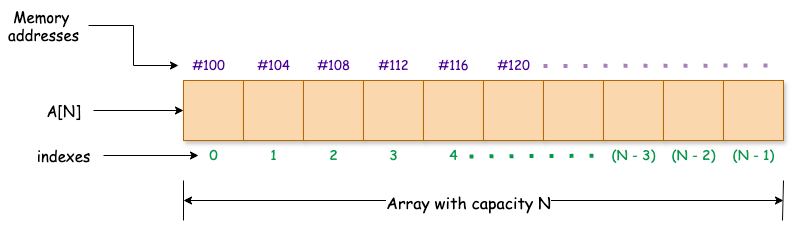
Figure 3 is an illustration of an array containing N
elements.
Since arrays in Java start from 0
th index. If you want to access the first element, you need to give A[0]
, and A[1]
for accessing the second element, and so on A[N-1]
to access the last element.
What happens if we do A[-100]
, A[N]
, and A[N+1]
? 🤔
You guessed it. We run into ArrayIndexOutOfBoundsException
.
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: Index 100 out of bounds for length 5
at array.ArrayIntroduction.main(ArrayIntroduction.java:20)
At most, we can access the last element of the array using A[N-1]
.
How to print array elements
A simple snippet to print the array elements from 0
to (N-1)
index.
public class PrintElements {
public static void main(String[] args) {
int[] A = {1, 2, 3, 4, 5};
int N = A.length;
for (int i = 0; i < N; i++) {
System.out.println(A[i]);
}
}
}
The time and space complexity to print these array elements are:
- Time complexity -
O(N)
- We iterated over all the array elements of sizeN
, so the time complexity is linear. - Space complexity -
O(1)
- No algorithmic memory is used here. We just used the inputA[]
memory, hence Constant time.
We have covered the basics of array data structures so far. In computer science, arrays are the building blocks for many other, more complex data structures.